Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1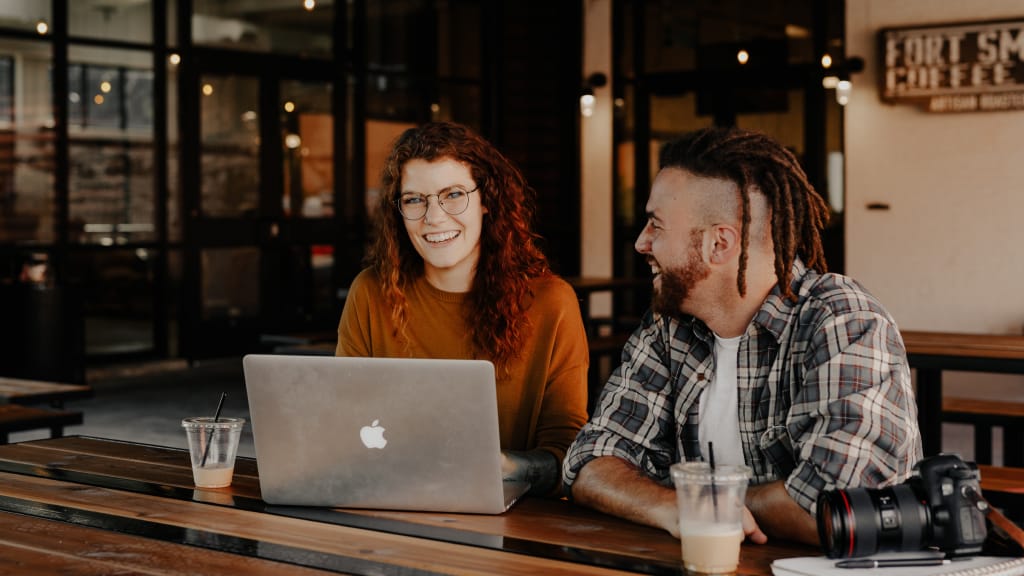
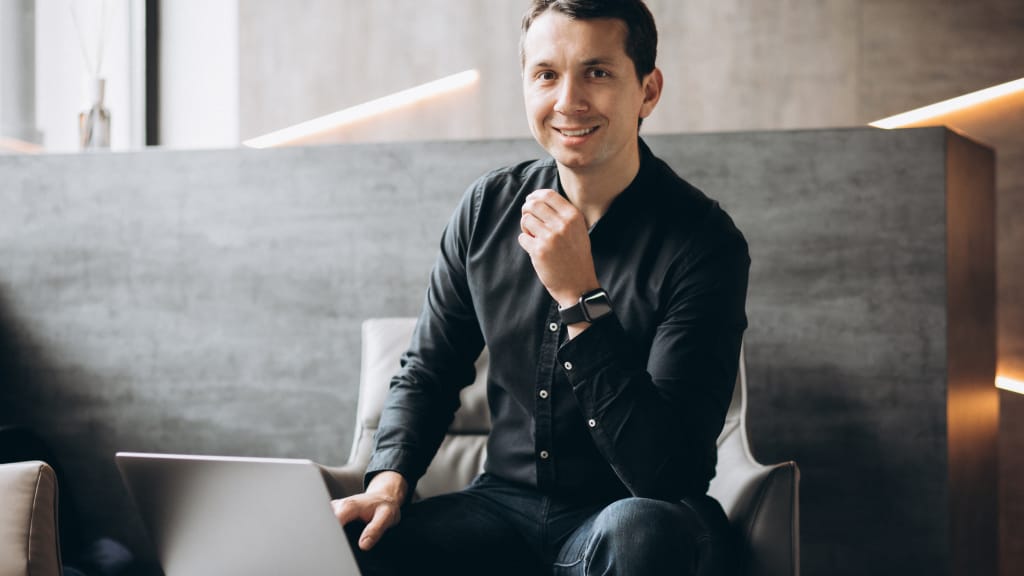
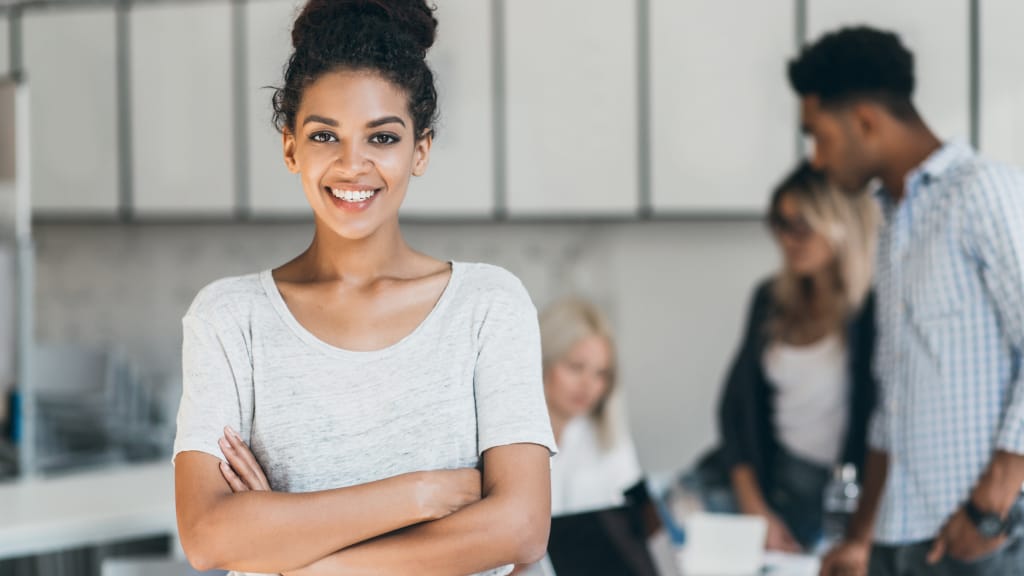
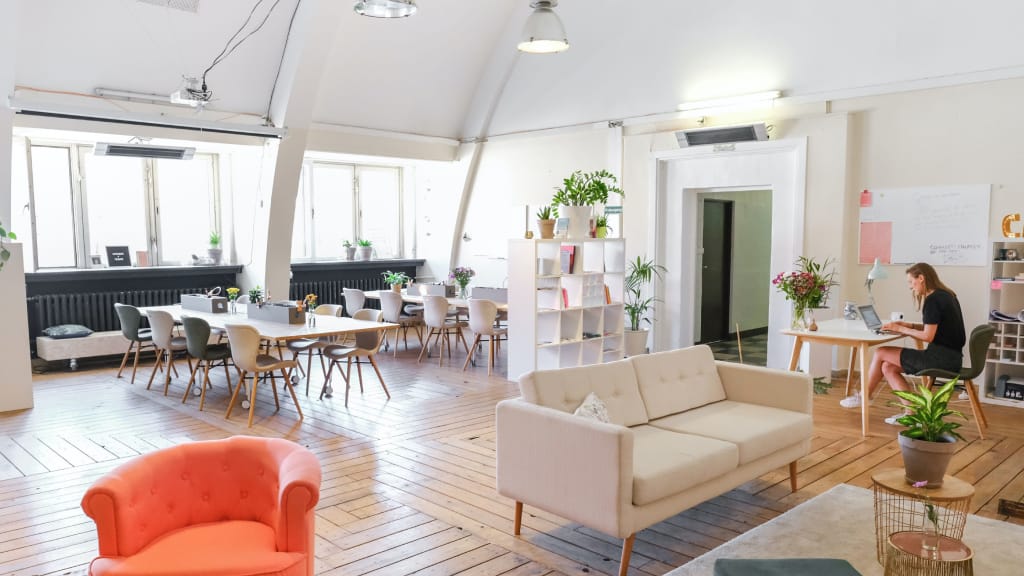
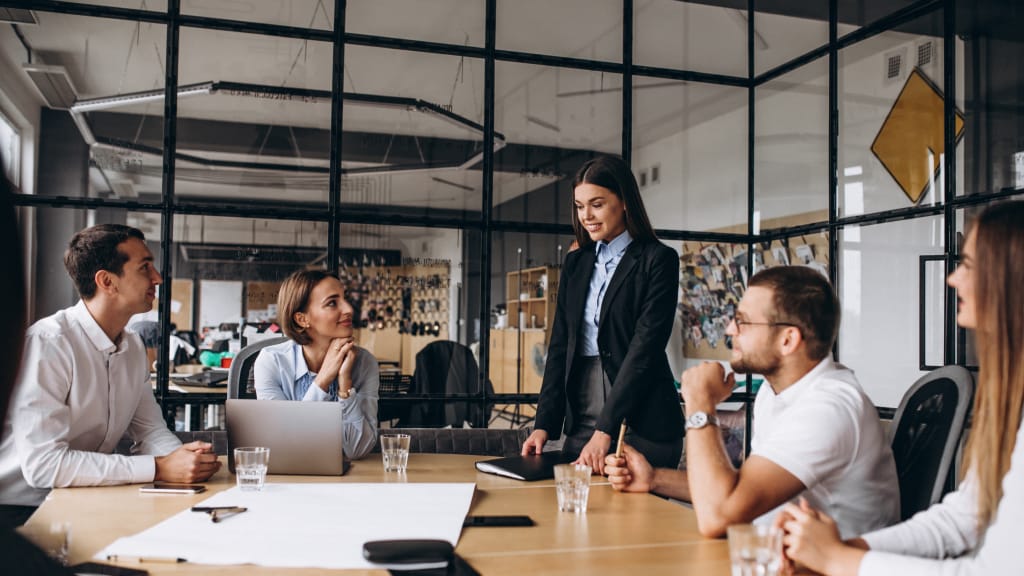
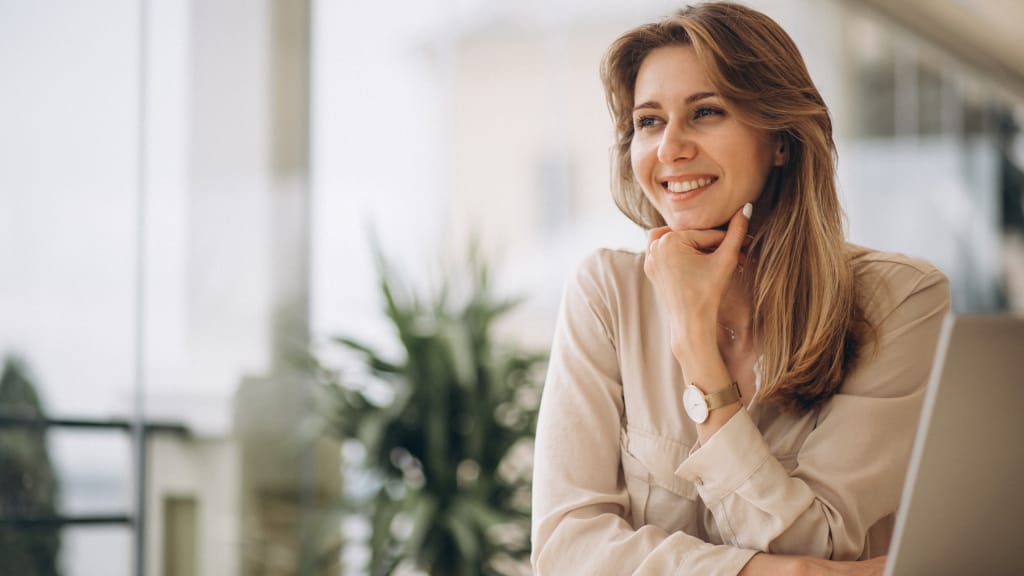
Java Access Modifiers: Controlling Access to Class Members
In Java, access modifiers are keywords that determine the accessibility of classes, methods, and variables within a program. Access modifiers help enforce encapsulation and control the visibility and availability of class members to other parts of the program. In this article, we will explore access modifiers in Java and provide examples to help you understand their usage.
The Four Access Modifiers
Java provides four access modifiers:
- public: The
public
modifier allows unrestricted access to a class, method, or variable. Public members are accessible from any part of the program, including other classes and packages. - private: The
private
modifier restricts access to a class, method, or variable within the same class. Private members are not accessible from outside the class, including subclasses and other packages. - protected: The
protected
modifier allows access to a class, method, or variable within the same class, subclasses, and the same package. Protected members are not accessible from other packages. - default (package-private): The default modifier, also known as package-private, does not use an explicit keyword. Members without any access modifier are accessible within the same package but not from outside the package.
Example: Access Modifiers in Action
Let's consider an example of a class that demonstrates the use of different access modifiers:
public class MyClass {
public int publicNumber;
private int privateNumber;
protected int protectedNumber;
int defaultNumber;
public void publicMethod() {
// Accessible from anywhere
}
private void privateMethod() {
// Accessible only within this class
}
protected void protectedMethod() {
// Accessible within this class, subclasses, and same package
}
void defaultMethod() {
// Accessible within the same package
}
}
In the above code, we define a class named MyClass
with different access modifiers applied to its members. The publicNumber
and publicMethod
have the public
modifier, allowing unrestricted access. The privateNumber
and privateMethod
have the private
modifier, restricting access to within the same class. The protectedNumber
and protectedMethod
have the protected
modifier, allowing access within the same class, subclasses, and the same package. The defaultNumber
and defaultMethod
do not have any explicit access modifier, making them accessible within the same package.
Accessing Class Members with Different Modifiers
To access class members with different access modifiers, you need to consider the visibility and the context of the access. Here's an example:
public class Main {
public static void main(String[] args) {
MyClass myObj = new MyClass();
myObj.publicNumber = 10; // Accessible from anywhere
myObj.publicMethod(); // Accessible from anywhere
// Cannot access myObj.privateNumber and myObj.privateMethod()
myObj.protectedNumber = 20; // Accessible within the same package
myObj.protectedMethod(); // Accessible within the same package
myObj.defaultNumber = 30; // Accessible within the same package
myObj.defaultMethod(); // Accessible within the same package
}
}
In the above code, we create an instance of the MyClass
class and access its members based on their respective access modifiers. We can access the publicNumber
and call the publicMethod
from anywhere. However, we cannot access the privateNumber
and call the privateMethod
as they are restricted to within the same class. The protectedNumber
and protectedMethod
can be accessed because they are within the same package. Similarly, we can access the defaultNumber
and call the defaultMethod
because they have the default (package-private) access within the same package.
Conclusion
Access modifiers in Java provide a way to control the accessibility of class members. In this article, we explored the four access modifiers: public
, private
, protected
, and default (package-private). We discussed how these modifiers restrict or allow access to class members based on their visibility and context. By effectively utilizing access modifiers, you can enforce encapsulation, control the visibility of class members, and create well-organized and maintainable code. Continuously practice using access modifiers and explore more advanced topics, such as access modifiers in inheritance and interfaces, to further enhance your object-oriented programming skills in Java.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses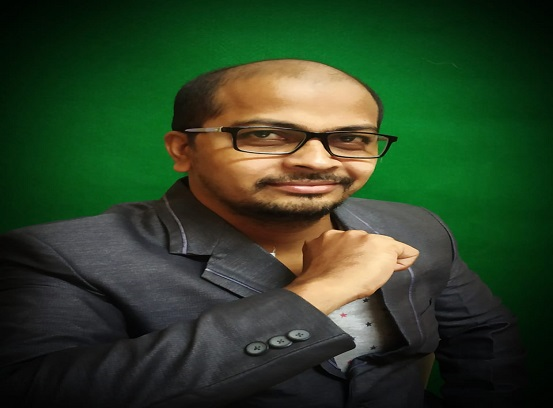
Most Popular Course topics
These are the most popular course topics among Software Courses for learners