Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1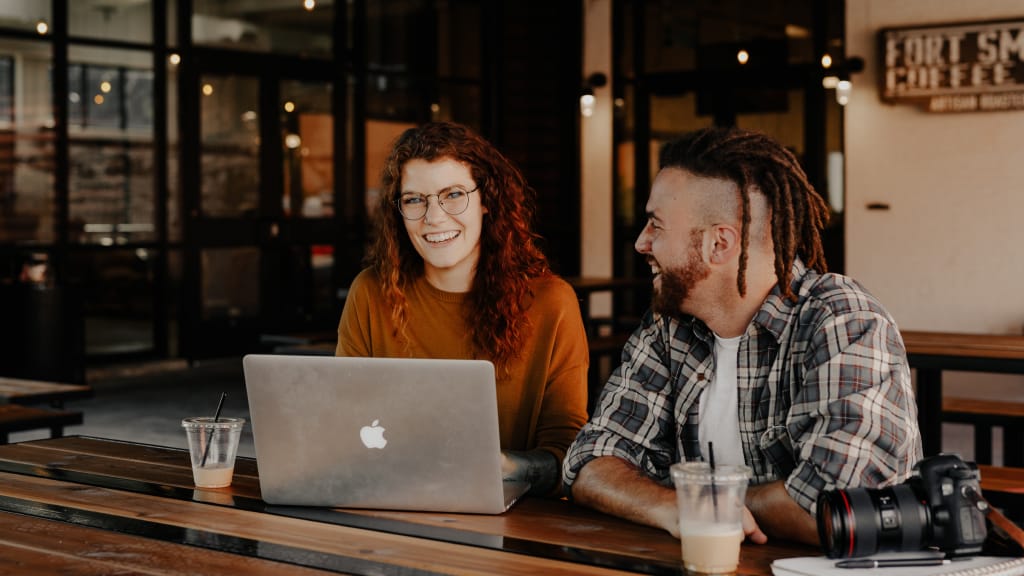
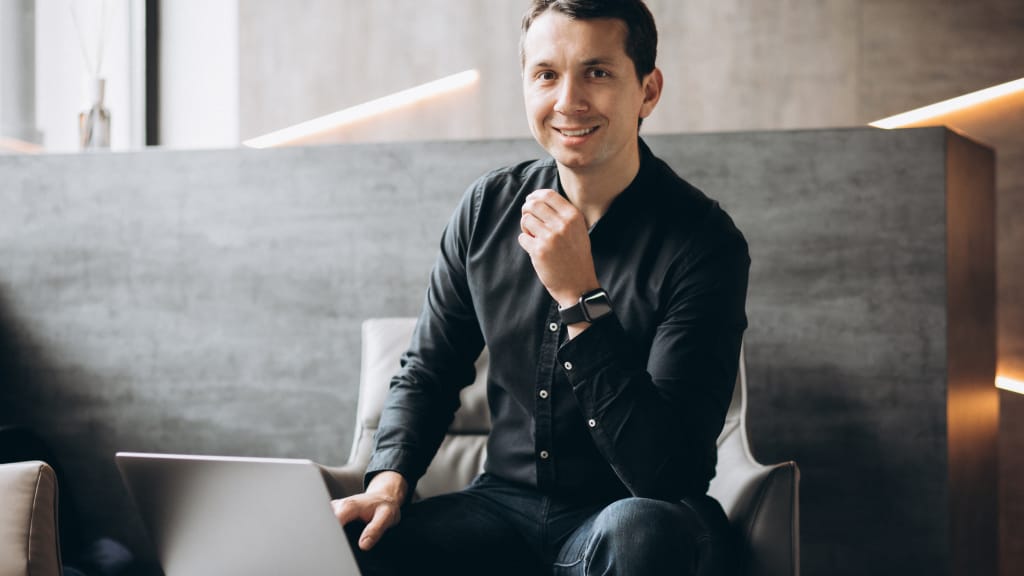
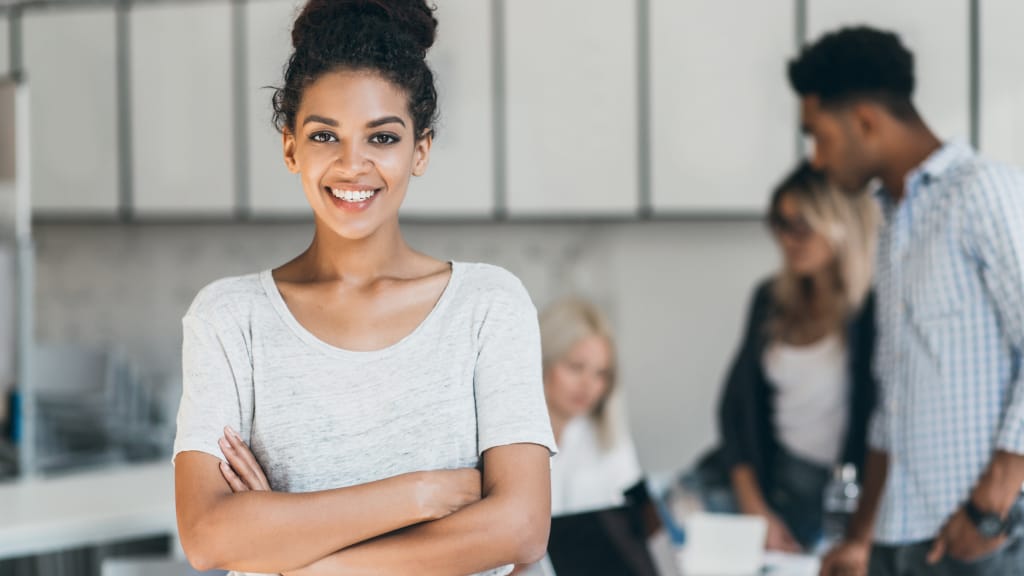
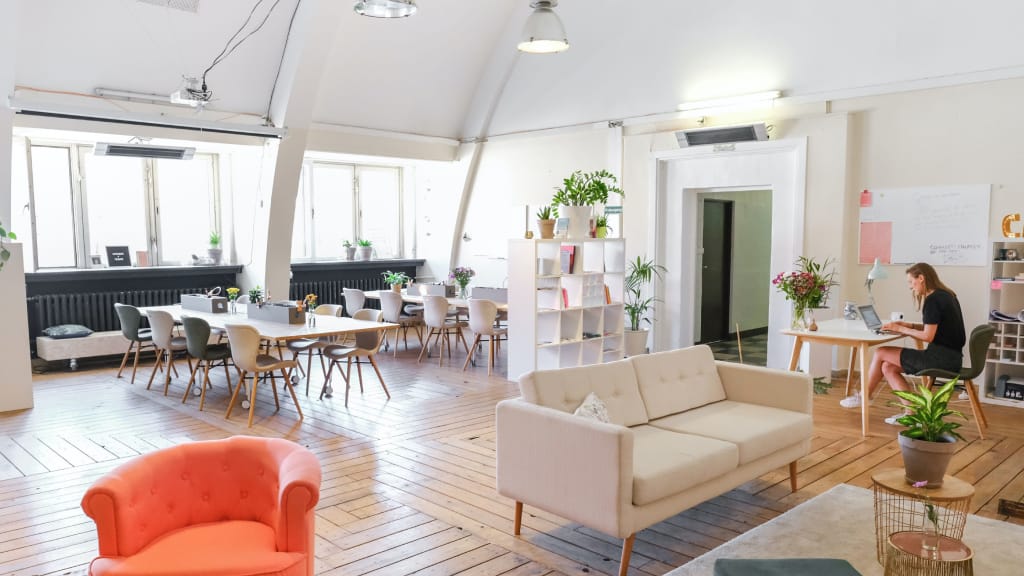
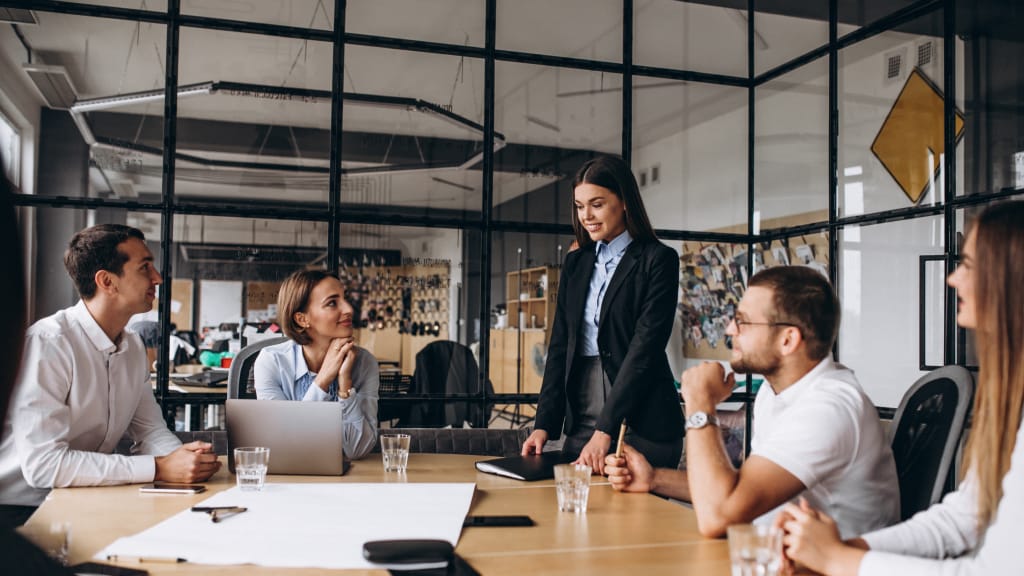
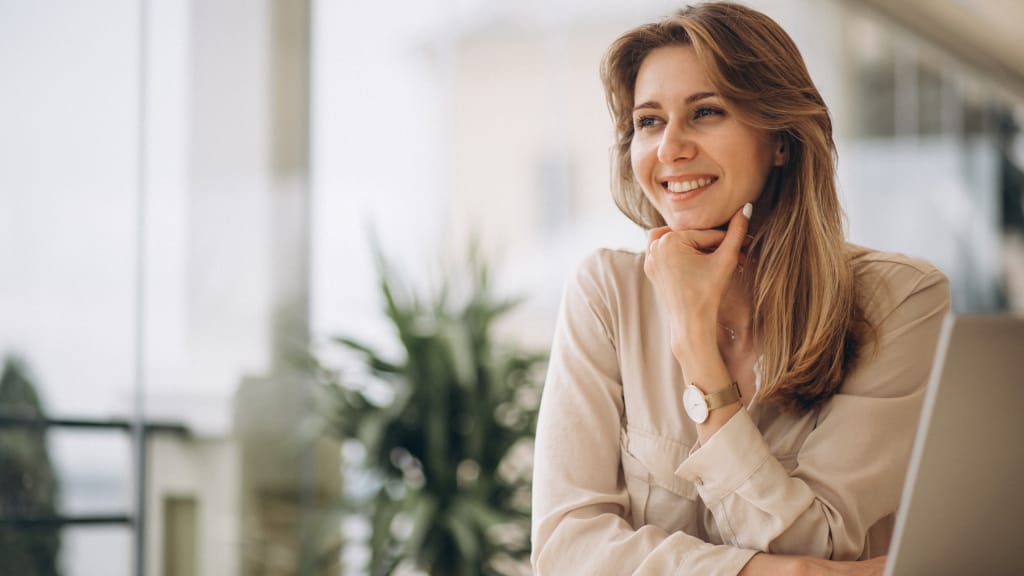
Django URLs: A Guide to URL Routing
Introduction
In Django, URL routing is an essential part of building web applications. It enables you to map URLs to specific views within your Django project. In this guide, we will explore how to define and configure URLs in Django with examples.
Step 1: Creating a Django Project
Before we dive into URLs, make sure you have a Django project set up. If you haven't created a Django project yet, you can refer to the previous guide on creating a Django project.
Step 2: Understanding URL Patterns
In Django, URL patterns define the mapping between URLs and views. Each URL pattern consists of a URL pattern string and a corresponding view function or class-based view. URL patterns are defined in the urls.py
file of your project or app.
Step 3: Creating URL Patterns
To create a URL pattern, open the urls.py
file in your project or app directory. Here's an example of a simple URL pattern that maps a URL to a view function:
from django.urls import path
from .views import my_view
urlpatterns = [
path('my-url/', my_view, name='my_view'),
]
In this example, we import the path
function from django.urls
and define a URL pattern for the path 'my-url/'
. When a user visits this URL, the my_view
function will be called.
Step 4: Capturing URL Parameters
You can capture parameters from the URL and pass them to your view functions. Use angle brackets (<
and >
) to capture parameters. Here's an example:
from django.urls import path
from .views import my_view
urlpatterns = [
path('my-url/<int:id>/', my_view, name='my_view'),
]
In this example, we capture an integer parameter named id
from the URL. The captured value will be passed as an argument to the my_view
function.
Step 5: Including URLs from Other Apps
If you have multiple apps in your Django project, you can include their URL patterns in the project's main URL configuration. Here's an example:
from django.urls import include, path
from myapp import urls as myapp_urls
urlpatterns = [
...
path('myapp/', include(myapp_urls)),
...
]
In this example, we import the include
function from django.urls
and include the URL patterns from the myapp
app.
Step 6: Using Named URLs
Django allows you to name your URL patterns, making it easier to reference them in your code. Here's an example:
from django.urls import path
from .views import my_view
urlpatterns = [
path('my-url/', my_view, name='my_view'),
]
In this example, we assign the name 'my_view'
to the URL pattern. You can use this name to reference the URL in your templates or Python code, allowing for more flexibility and maintainability.
Step 7: Testing URLs
To test your URLs, start the development server by running python manage.py runserver
in your terminal. Open your browser and navigate to the defined URLs to ensure they are correctly mapped to the desired views.
Conclusion
URL routing is a crucial aspect of Django development. By following this guide, you have learned how to define URL patterns, capture URL parameters, include URLs from other apps, and use named URLs. Understanding and properly configuring URLs will help you create a well-structured and accessible Django web application.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses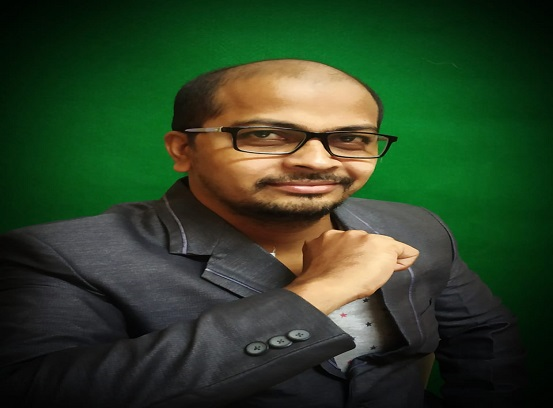
Most Popular Course topics
These are the most popular course topics among Software Courses for learners