Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1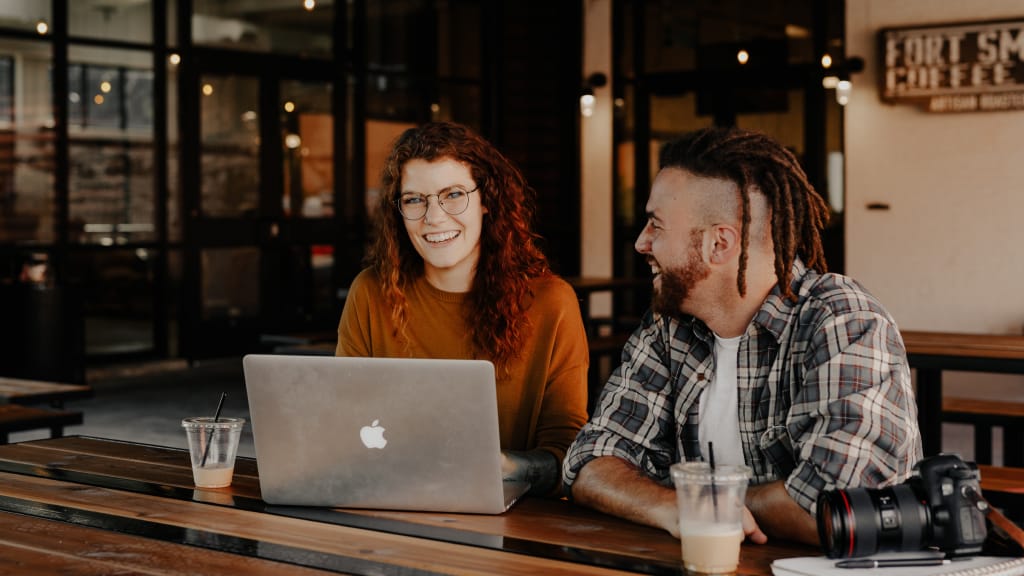
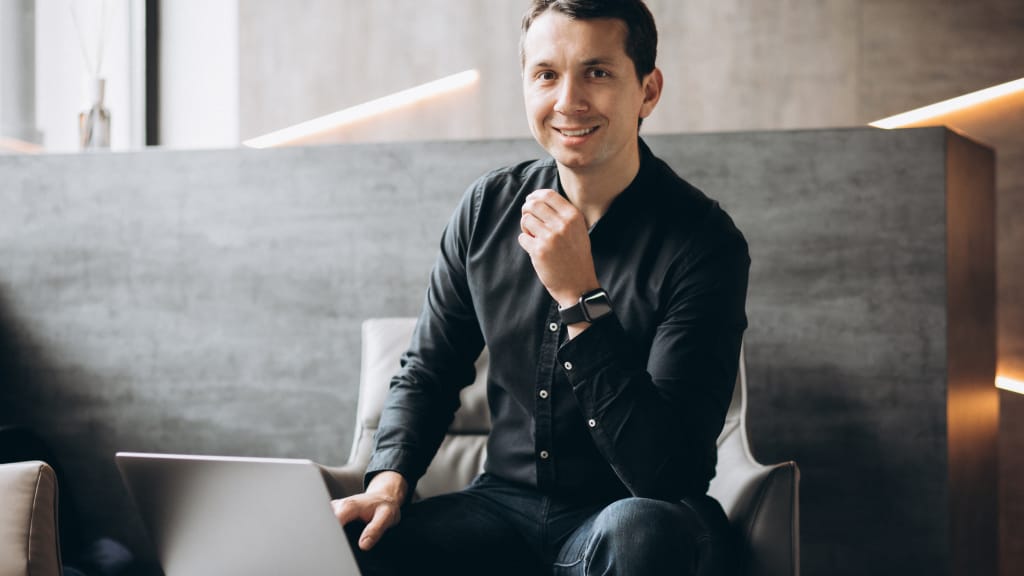
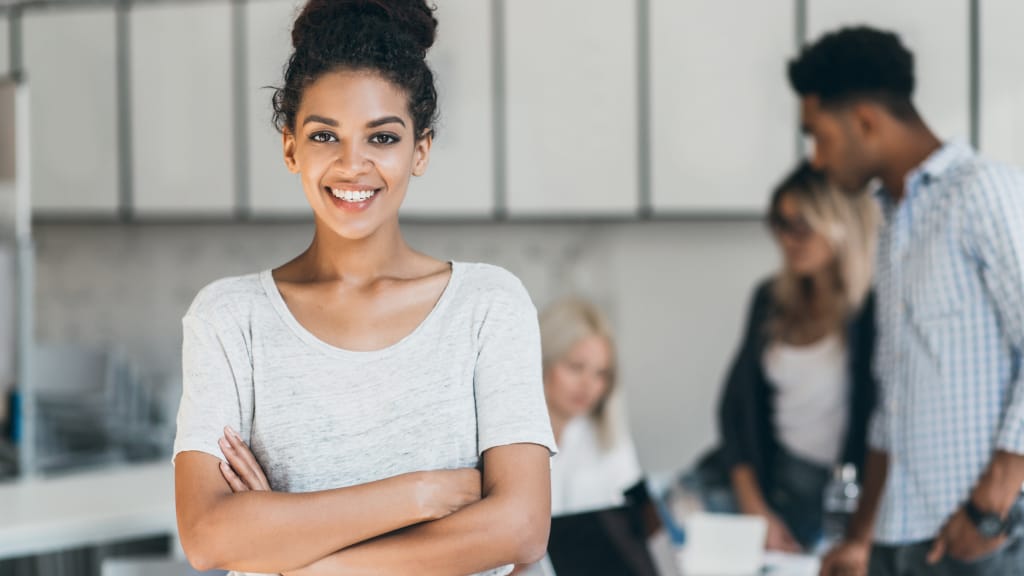
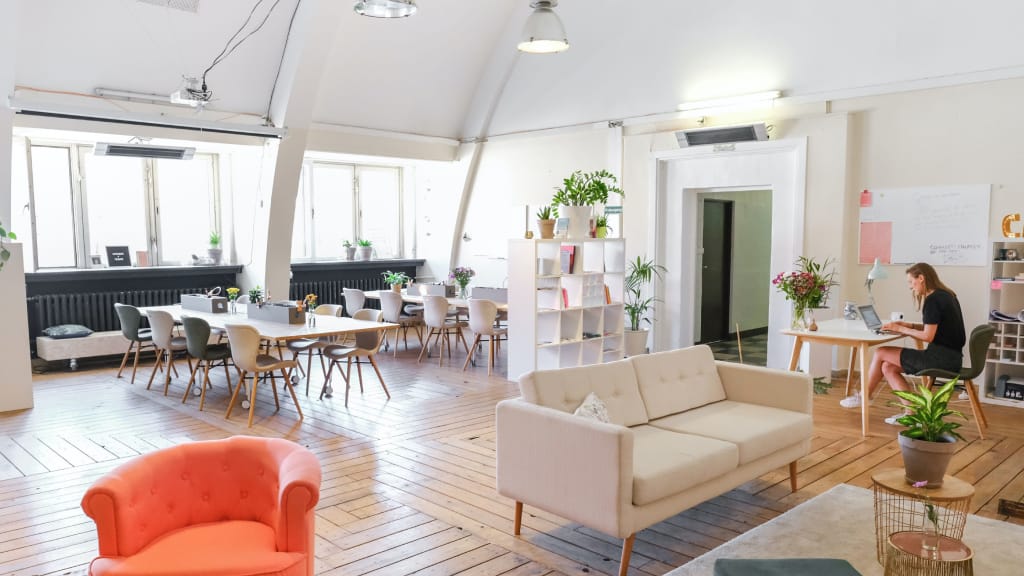
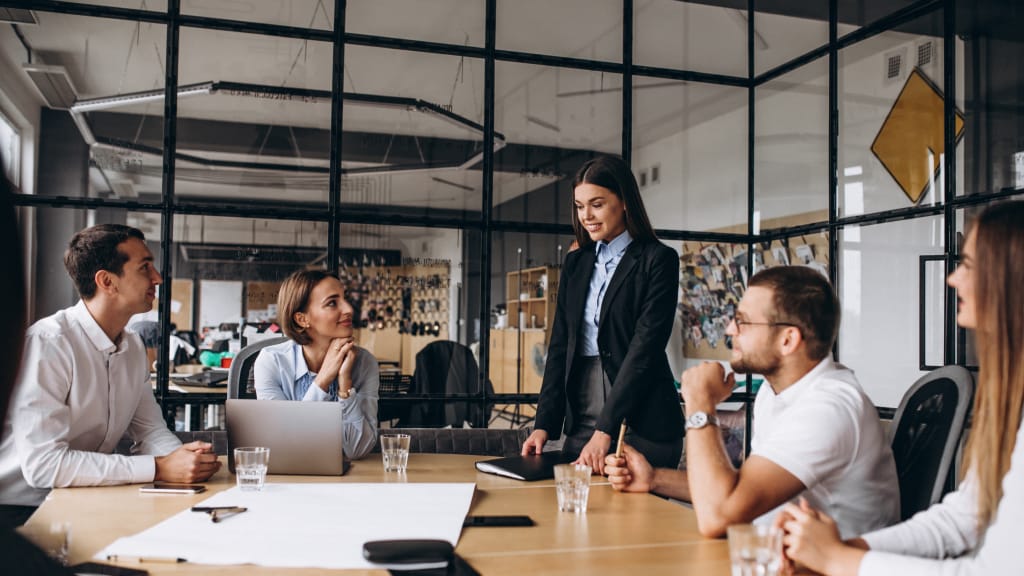
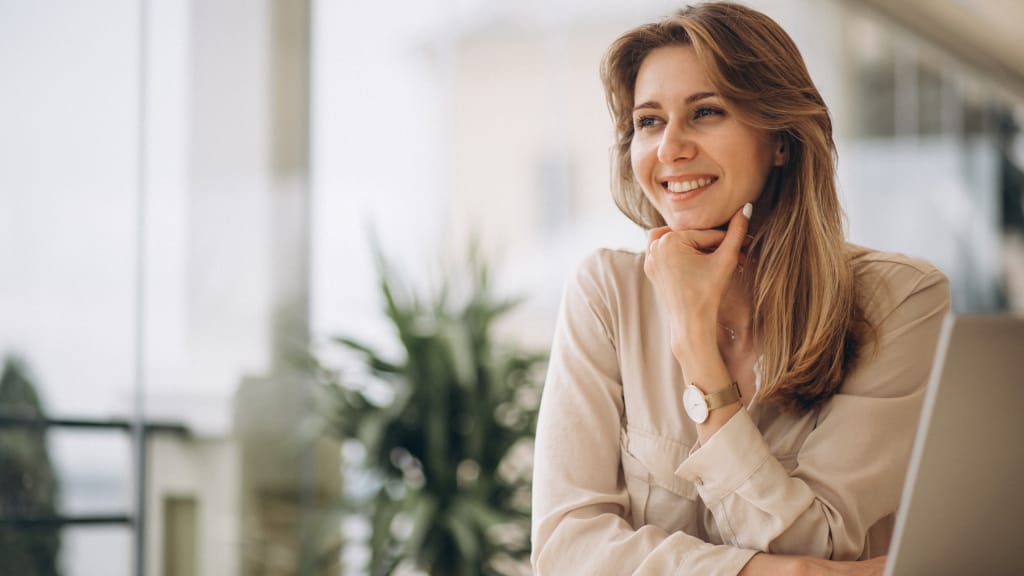
C++ Introduction
C++ is a powerful and versatile programming language that is widely used for developing a variety of applications. It was developed as an extension of the C programming language and provides additional features for object-oriented programming.
Here are a few key concepts and examples to help you understand the basics of C++:
1. Hello, World!
Let's start with a classic example: the "Hello, World!" program.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
2. Variables and Data Types
C++ provides several built-in data types, such as integers, floating-point numbers, characters, and booleans. Here's an example that demonstrates the usage of variables and data types:
#include <iostream>
int main() {
int age = 25;
float salary = 2500.50;
char grade = 'A';
bool isEmployed = true;
std::cout << "Age: " << age << std::endl;
std::cout << "Salary: " << salary << std::endl;
std::cout << "Grade: " << grade << std::endl;
std::cout << "Employed: " << isEmployed << std::endl;
return 0;
}
3. Control Flow
C++ supports various control flow statements, such as if-else, for loops, and while loops. Here's an example that uses a for loop to print numbers from 1 to 10:
#include <iostream>
int main() {
for (int i = 1; i <= 10; i++) {
std::cout << i << " ";
}
std::cout << std::endl;
return 0;
}
4. Functions
Functions in C++ allow you to break down your code into smaller, reusable units. Here's an example that defines a function to calculate the factorial of a number:
#include <iostream>
int factorial(int n) {
if (n == 0)
return 1;
else
return n * factorial(n - 1);
}
int main() {
int number = 5;
int result = factorial(number);
std::cout << "Factorial of " << number << " is " << result << std::endl;
return 0;
}
These examples provide a glimpse into the world of C++ programming. With C++, you can build complex software systems, develop games, create efficient algorithms, and much more. It's a language that offers both high-level abstractions and low-level control, making it suitable for a wide range of applications.
Remember, practice is key to mastering any programming language. Explore more C++ resources, experiment with code, and keep learning!
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses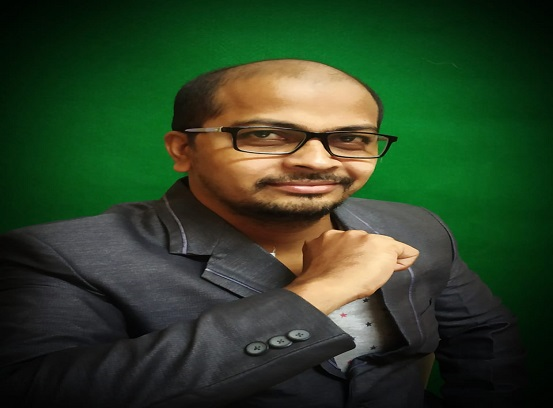
Most Popular Course topics
These are the most popular course topics among Software Courses for learners